First give a meaningful name to our function. Say max function is used to find maximum between two numbers. Second, we need to find maximum between two numbers. Hence, the function must accept two parameters of int type say, max (int num1, int num2). Finally, the function should return maximum among given two numbers. We would like to show you a description here but the site won’t allow us. C/C Code Generation Generate C and C code using MATLAB® Coder™. Usage notes and limitations: If you specify an empty array for the second argument in order to supply dim or nanflag, the second argument must be of fixed-size and of dimension 0 -by- 0. Returns the minimum finite value representable by the numeric type T. For floating-point types with denormalization, min returns the minimum positive normalized value.Note that this behavior may be unexpected, especially when compared to the behavior of min for integral types.
- C++ Math Min
- C++ Min In Vector
- Min In C++
- C++ Minimum Value
- Min In C++ Vector
- Find Max And Min In C++
- C++ Minimum
Enumerable.Min is extension method from System.Linq namespace. It returns minimal value of numeric collection.
Min for Numeric Types

C++ Math Min
Gets minimal number from list of integer numbers.
Gets minimal number from list of decimal numbers.
Calling Min on empty collection throws exception.
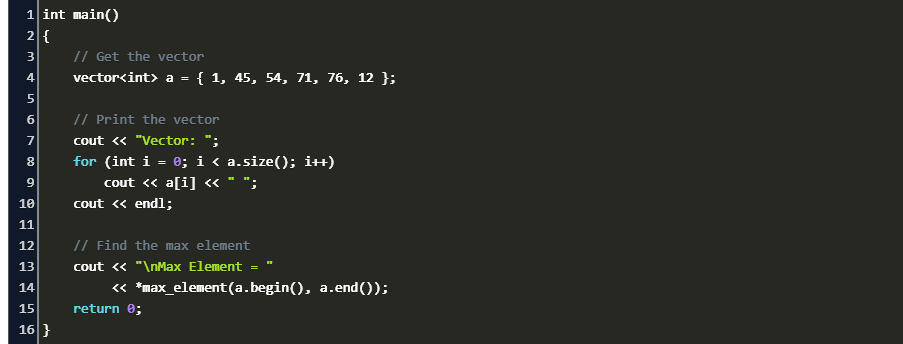

Min for Nullable Numeric Types
Gets minimal number from list of nullable integers.
Returns null if the collection contains only null values.
Returns null if the collection is empty.
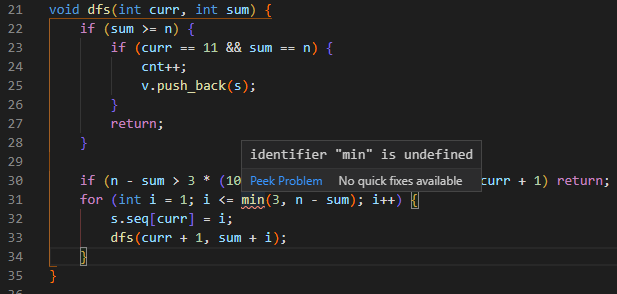
Min with Selector
C++ Min In Vector
This example gets length of the longest string.
Min for Types Implementing IComparable
LINQ method Min can be used also on collection of custom type (not numeric type like int or decimal). The custom type have to implement IComparable interface.
Lets have custom type Money which implements IComparable.
And this is usage of Min method on the Money collection.
Min with Query Syntax
LINQ query expression to get minimal item in the collection.
Min In C++
LINQ query expression to get minimal item which matches specified predicate.
LINQ query expression to get minimal string length using selector.
Min with Group By
This example shows how to get minimal value per group. Lets have players. Each player belongs to a team and have a score. Team best score is minimal score of players in a team.
Min Implementation
This is .NET Framework implementation of Enumerable.Min method for collection of numeric type (in this case IEnumerable<int>
). Note that it throws the exception for an empty collection on the last line (throwError.NoElements();
)).
C++ Minimum Value
This is .NET Framework implementation of Enumerable.Min method for collection of nullable numeric type (in this case IEnumerable<int?>
). Note that it returns null for an empty collection (int? value = null;
). Also notice that it returns null if all values in the collection are null.
Min In C++ Vector
See also
- MSDN Enumerable.Min - .NET Framework documentation
- LINQ Aggregation Methods
- LINQ Sum - gets sum of numeric collection
- LINQ Max - gets maximal item from collection
- LINQ Min - gets minimal item from collection
- LINQ Count - counts number of items in a collection (result type is Int32)
- LINQ LongCount - counts number of items in a collection (result type is Int64)
- LINQ Average - computes average value of numeric collection
- LINQ Aggregate - applies aggregate function to a collection
- C# List - illustrative examples of all List<T> methods
- C# foreach - how foreach and IEnumerable works debuggable online
- Deliver and maintain services, like tracking outages and protecting against spam, fraud, and abuse
- Measure audience engagement and site statistics to understand how our services are used
Find Max And Min In C++
- Improve the quality of our services and develop new ones
- Deliver and measure the effectiveness of ads
- Show personalized content, depending on your settings
- Show personalized or generic ads, depending on your settings, on Google and across the web
C++ Minimum
Click “Customize” to review options, including controls to reject the use of cookies for personalization and information about browser-level controls to reject some or all cookies for other uses. You can also visit g.co/privacytools anytime.
